Next: Linux Thread Implementation
Up: SYSTEMS PROGRAMMING LABORATORY V
Previous: Examples&Exercises:
Contents
- If the threads work too quickly, the queue of jobs will empty and the threads will exit.
- What we might like instead is a mechanism for blocking the threads when the queue empties until new jobs become available.
- A semaphore is a counter (non-negative integer) that can be used to synchronize multiple threads. As with a mutex, Linux guarantees that checking or modifying the value of a semaphore can be done safely, without creating a race condition.
- A semaphore supports two basic operations:
- A wait (down) operation decrements the value of the semaphore by 1. If the value is already zero, the operation blocks until the value of the semaphore becomes positive (due to the action of some other thread). When the semaphore's value becomes positive, it is decremented by 1 and the wait operation returns.
- A post (up) operation increments the value of the semaphore by 1. If the semaphore was previously zero and other threads are blocked in a wait operation on that semaphore, one of those threads is unblocked and its wait operation completes (which brings the semaphore's value back to zero).
- A semaphore is represented by a sem_t variable.
- initialize it using the sem_init function, passing a pointer to the sem_t variable.
- the second parameter should be zero.
- the third parameter is the semaphore's initial value.
- to deallocate it with sem_destroy.
- to wait on a semaphore, use sem_wait.
- to post to a semaphore, use sem_post.
- a nonblocking wait function, sem_trywait, is also provided.
- to retrieve the current value of a semaphore, sem_getvalue, (to do this could lead to a race condition).
- The following http://siber.cankaya.edu.tr/SystemsProgramming/cfiles/job-queue3.ccode (see Fig. 5.5.1) controls the queue with a semaphore.
Figure 5.10:
Job Queue Controlled by a Semaphore
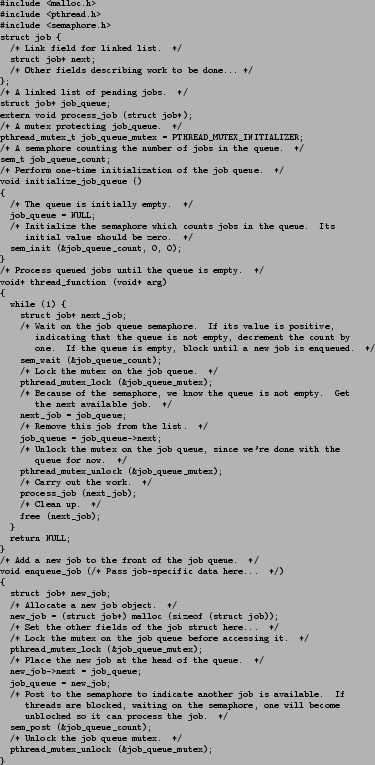 |
- If the semaphore's value is zero, indicating that the queue is empty, the thread will simply block until the semaphore s value becomes positive, indicating that a job has been added to the queue.
Next: Linux Thread Implementation
Up: SYSTEMS PROGRAMMING LABORATORY V
Previous: Examples&Exercises:
Contents
Cem Ozdogan
2007-05-16